Writing algorithms, or instructions, to a computer to do a certain activity is called coding. Programming languages are used by developers to connect with computers.
A similar set of syntactic and semantic norms underpins computer languages as do natural languages like English, Russian, or Quechua. These conventions serve as the foundation for fundamental communication. Although natural languages are more sophisticated, flexible, and dynamic, these traits are also relevant to computer languages to a more limited level.
Even the most basic algorithm may be written in a variety of ways. While some flexibility is ideal while writing code, when several people are working on it, it might jeopardize productivity and clear communication.
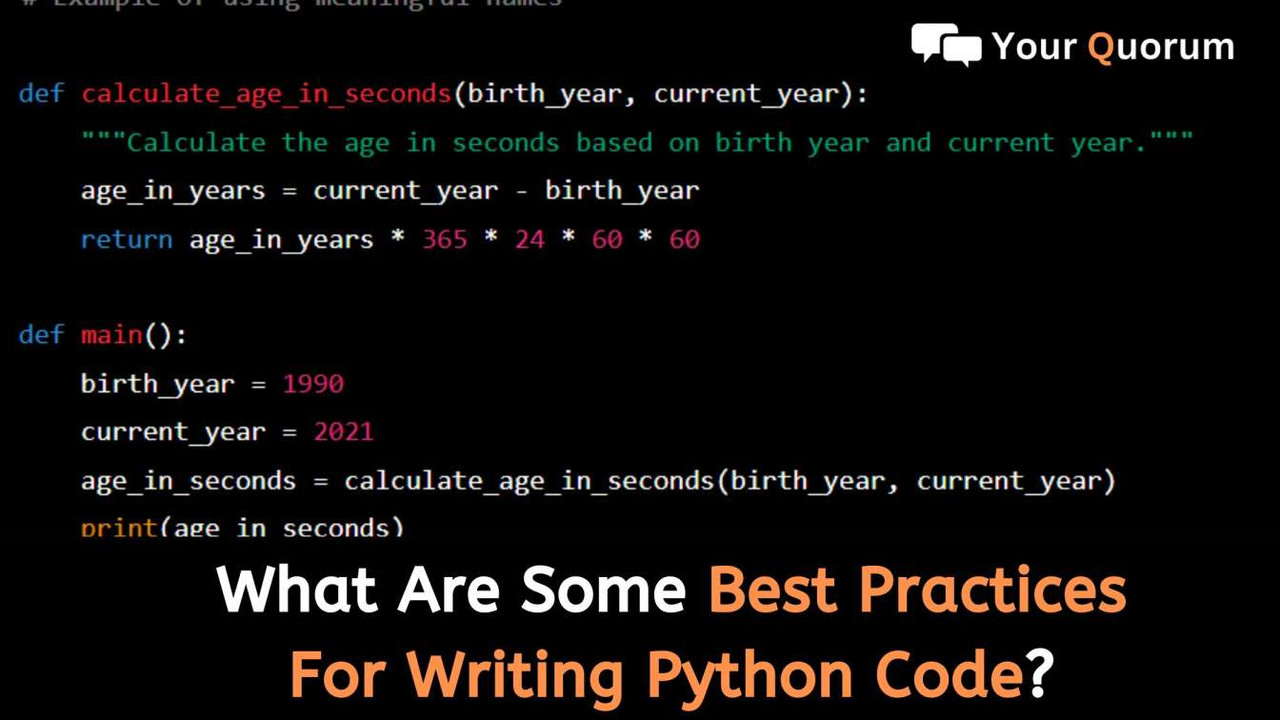
Readability is therefore a vital component of developing code. The majority of computer languages have coding standards in place to make sure developers are working together. These guides offer recommendations and best practices for writing scalable, readable, and maintainable code.
Read Also: How many important libraries are there in Python?
What is PEP 8?
"Much more frequently than not, code is read than written. Writing code should always aim to make it as readable as possible. Guido van Rossum, the person who created Python
A style guide for Python programming is called PEP 8. Guido van Rossum, Barry Warsaw, and Nick Coghlan wrote it in 2001, and it offers some advice on how to write code that is easier to understand and more consistent. Everything is covered, including the maximum character count for a line and how variables should be named.
Best Practices for Python
1. Python code quality best practices
You may improve your job chances and make a difference by writing code that is well-organized and legible. For novices, learning to code may appear like a mechanical and difficult procedure, but in actuality, coding is an art.
There are several strategies you may take to boost code quality in Python. Some of the more relevant ones are included below.
The tyranny of indentation
The spaces that appear at the start of a code line are known as indentation. In Python, indentation is required, although in other programming languages it is only a readability tool. Python opens a piece of code using indentation. More exactly, 4 consecutive spaces each indentation level, as illustrated in the following code:
for number in [1,2,3,4]:
if number % 2 == 0:
print(number)
else:
continue
2. Python logging best practices
Software logging is a way to monitor events that occur during software operation. Logging becomes an essential tool for creating, troubleshooting, executing, and monitoring the performance of applications, particularly as they get larger and more sophisticated.
Since version 2.3, the logging module has been a part of the Python Standard Library, serving to handle various logging patterns. It is fully documented in PEP 282, and for most Python developers, it is the first package they use for logging.
3. Best practices for Python commenting
When creating annotations for readers of our code in the future, comments are crucial. Despite the fact that it is challenging to provide the appropriate comments for the code, we may adhere to the following rules:
It is worse to have a comment than not to have one that goes against the code. To prevent inconsistencies, it is crucial to update both the code and the comments.
Sentences must be capitalized starting with the first letter in the comment.
Try using English while writing comments. It is advised that comments be written in English, although everyone is free to contribute in any language they see suitable.
4. Python docstring best practices
A string literal that appears as the first sentence in the definition of a module, function, class, or method is known as a docstring. Usually, the code documentation is generated automatically using a documentation string. Consequently, a docstring may be retrieved at runtime by utilizing the object's obj.__doc__ special property.
Docstrings are always surrounded by triple double quotes (""") for consistency's sake.
There are two sorts of docstrings: one-liners and multi-line docstrings. Single words are for the most apparent situations. Really, they ought to fit on a single line. A one-line docstring in the multiply() method is demonstrated in the example that follows:
def multiply(x, y):
""" Return the product of two numbers """
result = x * y
return result
5. Best practices for Python documentation
Documentation is meant to teach a community of potential customers how to use your product, whereas comments are crucial for other developers you work with to comprehend your code. This is a crucial stage because, regardless of how amazing your program is, users won't use it if the documentation is poor quality or, worse, nonexistent.
It's usually a good idea to include a README file, particularly if you're working on a new project. These files, which provide basic information for both users and project maintainers, are typically the primary point of entry for readers of your code.
Writing algorithms, or instructions, to a computer to do a certain activity is called coding. Programming languages are used by developers to connect with computers.
A similar set of syntactic and semantic norms underpins computer languages as do natural languages like English, Russian, or Quechua. These conventions serve as the foundation for fundamental communication. Although natural languages are more sophisticated, flexible, and dynamic, these traits are also relevant to computer languages to a more limited level.
Even the most basic algorithm may be written in a variety of ways. While some flexibility is ideal while writing code, when several people are working on it, it might jeopardize productivity and clear communication.
Readability is therefore a vital component of developing code. The majority of computer languages have coding standards in place to make sure developers are working together. These guides offer recommendations and best practices for writing scalable, readable, and maintainable code.
Read Also: How many important libraries are there in Python?
What is PEP 8?
"Much more frequently than not, code is read than written. Writing code should always aim to make it as readable as possible. Guido van Rossum, the person who created Python
A style guide for Python programming is called PEP 8. Guido van Rossum, Barry Warsaw, and Nick Coghlan wrote it in 2001, and it offers some advice on how to write code that is easier to understand and more consistent. Everything is covered, including the maximum character count for a line and how variables should be named.
Best Practices for Python
1. Python code quality best practices
You may improve your job chances and make a difference by writing code that is well-organized and legible. For novices, learning to code may appear like a mechanical and difficult procedure, but in actuality, coding is an art.
There are several strategies you may take to boost code quality in Python. Some of the more relevant ones are included below.
The tyranny of indentation
The spaces that appear at the start of a code line are known as indentation. In Python, indentation is required, although in other programming languages it is only a readability tool. Python opens a piece of code using indentation. More exactly, 4 consecutive spaces each indentation level, as illustrated in the following code:
2. Python logging best practices
Software logging is a way to monitor events that occur during software operation. Logging becomes an essential tool for creating, troubleshooting, executing, and monitoring the performance of applications, particularly as they get larger and more sophisticated.
Since version 2.3, the logging module has been a part of the Python Standard Library, serving to handle various logging patterns. It is fully documented in PEP 282, and for most Python developers, it is the first package they use for logging.
3. Best practices for Python commenting
When creating annotations for readers of our code in the future, comments are crucial. Despite the fact that it is challenging to provide the appropriate comments for the code, we may adhere to the following rules:
It is worse to have a comment than not to have one that goes against the code. To prevent inconsistencies, it is crucial to update both the code and the comments.
Sentences must be capitalized starting with the first letter in the comment.
Try using English while writing comments. It is advised that comments be written in English, although everyone is free to contribute in any language they see suitable.
4. Python docstring best practices
A string literal that appears as the first sentence in the definition of a module, function, class, or method is known as a docstring. Usually, the code documentation is generated automatically using a documentation string. Consequently, a docstring may be retrieved at runtime by utilizing the object's obj.__doc__ special property.
Docstrings are always surrounded by triple double quotes (""") for consistency's sake.
There are two sorts of docstrings: one-liners and multi-line docstrings. Single words are for the most apparent situations. Really, they ought to fit on a single line. A one-line docstring in the multiply() method is demonstrated in the example that follows:
5. Best practices for Python documentation
Documentation is meant to teach a community of potential customers how to use your product, whereas comments are crucial for other developers you work with to comprehend your code. This is a crucial stage because, regardless of how amazing your program is, users won't use it if the documentation is poor quality or, worse, nonexistent.
It's usually a good idea to include a README file, particularly if you're working on a new project. These files, which provide basic information for both users and project maintainers, are typically the primary point of entry for readers of your code.