Problems Building a MySQL Table Using PHP
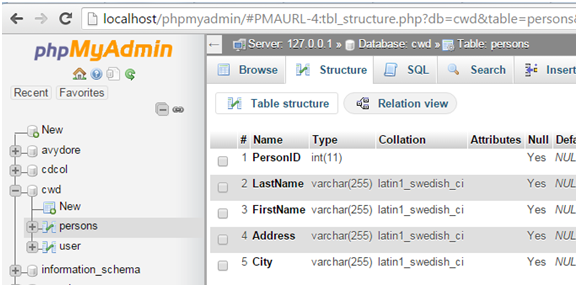
You're not alone if you have problems trying to create a table in MySQL using PHP. These typical mistakes and fixes will help you troubleshoot:
1. Review SQL syntax here: Check your SQL query for accuracy. A standard CREATE TABLE statement like this:
CREATE TABLE your_table_name (
id INT AUTO_INCREMENT PRIMARY KEY,
column1 VARCHAR(255) NOT NULL,
column2 INT
);
Check your SQL query for typos or syntactic mistakes.
2. Verify Database Connection: Check to be sure your PHP script is correctly connected to the MySQL database. Search for any connection mistakes using the mysqli_connect() function:
$conn = mysqli_connect("localhost", "username", "password", "database");
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
3. Error Reporting: Turn on error reporting to obtain more specific error notes. Line these at the top of your script:
mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT);
4. Check for Existing Tables: Make sure you are not trying to create an existing table. Use IF NOT EXISEST in your search to steer clear of conflicts:
CREATE TABLE IF NOT EXISTS your_table_name (
id INT AUTO_INCREMENT PRIMARY KEY,
column1 VARCHAR(255) NOT NULL,
column2 INT
);
5. Permissions: Make that the MySQL user you are working with has the required permissions to create tables. Examine your MySQL user rights and, if needed, change.
6. Review PHP logs to find any further hints or error messages suggesting what went wrong.
By systematically addressing these areas, you should be able to resolve most issues related to table creation in MySQL with PHP.
Related: How can I refresh a jQuery DataTable without making a database call?
Problems Building a MySQL Table Using PHP
You're not alone if you have problems trying to create a table in MySQL using PHP. These typical mistakes and fixes will help you troubleshoot:
1. Review SQL syntax here: Check your SQL query for accuracy. A standard CREATE TABLE statement like this:
Check your SQL query for typos or syntactic mistakes.
2. Verify Database Connection: Check to be sure your PHP script is correctly connected to the MySQL database. Search for any connection mistakes using the mysqli_connect() function:
3. Error Reporting: Turn on error reporting to obtain more specific error notes. Line these at the top of your script:
4. Check for Existing Tables: Make sure you are not trying to create an existing table. Use IF NOT EXISEST in your search to steer clear of conflicts:
5. Permissions: Make that the MySQL user you are working with has the required permissions to create tables. Examine your MySQL user rights and, if needed, change.
6. Review PHP logs to find any further hints or error messages suggesting what went wrong.
By systematically addressing these areas, you should be able to resolve most issues related to table creation in MySQL with PHP.
Related: How can I refresh a jQuery DataTable without making a database call?