Flattening an array in JavaScript involves evolving a multi-dimensional array (an array that includes other arrays as its elements) into a one-dimensional array. This method is helpful when you want to simplify the structure of data, making it simpler to analyze or modify. There are various techniques to flatten arrays in JavaScript, each with its own merits and use cases. Let’s learn some of the most common ways.
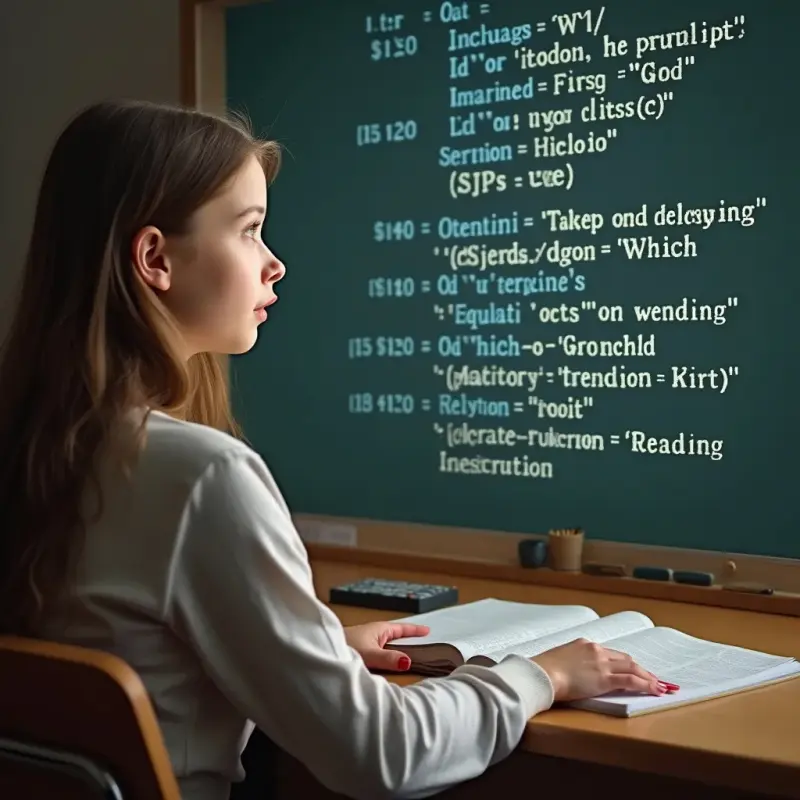
1. Using Array.prototype.flat()
The easiest and most modern approach to flatten an array is to use the flat() function, introduced in ECMAScript 2019 (ES10). This function creates a new array with all sub-array elements concatenated into it recursively up to a given depth.
const nestedArray = [1, [2, [3, 4]], 5];
const flattenedArray = nestedArray.flat(2); // Flatten to depth 2
console.log(flattenedArray); // Output: [1, 2, 3, 4, 5
By default, flat() flattens to a depth of 1, but you may deliver a different depth if needed.
2. Using Array.prototype.reduce()
Another method to flatten an array is by using the reduce() function in combination with the concat() method. This method enables for a custom flattening processes.
const nestedArray = [1, [2, [3, 4]], 5];
const flatten = (arr) => arr.reduce((acc, val) => acc.concat(Array.isArray(val) ? flatten(val) : val), []);
const flattenedArray = flatten(nestedArray);
console.log(flattenedArray); // Output: [1, 2, 3, 4, 5
This function works by repeatedly concatenating each value to the accumulator, verifying whether the value is an array.
Read More: Which is the most used design pattern in JavaScript?
3. Using the Spread Operator
You may also flatten an array with the spread operator (...). This approach works well for arrays that are merely one level deep.
const nestedArray = [1, [2, 3], 4];
const flattenedArray = [].concat(...nestedArray);
console.log(flattenedArray); // Output: [1, 2, 3, 4
4. Using a Recursive Function
If you want total of control over the flattening process, you may build a recursive function that flattens the array to any depth.
function flattenArray(arr) {
let result = [];
arr.forEach(item => {
if (Array.isArray(item)) {
result = result.concat(flattenArray(item)); // Recursive call
} else {
result.push(item);
}
});
return result;
}
const nestedArray = [1, [2, [3, 4]], 5];
const flattenedArray = flattenArray(nestedArray);
console.log(flattenedArray); // Output: [1, 2, 3, 4, 5
5. Using Libraries
For more complex applications, try using libraries like Lodash. The _.flattenDeep function may flatten arrays of any depth easily.
const _ = require('lodash');
const nestedArray = [1, [2, [3, 4]], 5];
const flattenedArray = _.flattenDeep(nestedArray);
console.log(flattenedArray); // Output: [1, 2, 3, 4, 5
Flattening arrays in JavaScript may be performed by many approaches, each suitable for particular purposes and complications. The flat() technique is the most easy for current applications, but recursive functions and libraries allow greater flexibility for deeper or more complicated structures. Understanding these techniques may help you effectively manage nested data in your JavaScript applications.
Flattening an array in JavaScript involves evolving a multi-dimensional array (an array that includes other arrays as its elements) into a one-dimensional array. This method is helpful when you want to simplify the structure of data, making it simpler to analyze or modify. There are various techniques to flatten arrays in JavaScript, each with its own merits and use cases. Let’s learn some of the most common ways.
1. Using
Array.prototype.flat()
The easiest and most modern approach to flatten an array is to use the flat() function, introduced in ECMAScript 2019 (ES10). This function creates a new array with all sub-array elements concatenated into it recursively up to a given depth.
By default, flat() flattens to a depth of 1, but you may deliver a different depth if needed.
2. Using
Array.prototype.reduce()
Another method to flatten an array is by using the reduce() function in combination with the concat() method. This method enables for a custom flattening processes.
This function works by repeatedly concatenating each value to the accumulator, verifying whether the value is an array.
Read More: Which is the most used design pattern in JavaScript?
3. Using the Spread Operator
You may also flatten an array with the spread operator (...). This approach works well for arrays that are merely one level deep.
4. Using a Recursive Function
If you want total of control over the flattening process, you may build a recursive function that flattens the array to any depth.
5. Using Libraries
For more complex applications, try using libraries like Lodash. The _.flattenDeep function may flatten arrays of any depth easily.
Flattening arrays in JavaScript may be performed by many approaches, each suitable for particular purposes and complications. The flat() technique is the most easy for current applications, but recursive functions and libraries allow greater flexibility for deeper or more complicated structures. Understanding these techniques may help you effectively manage nested data in your JavaScript applications.